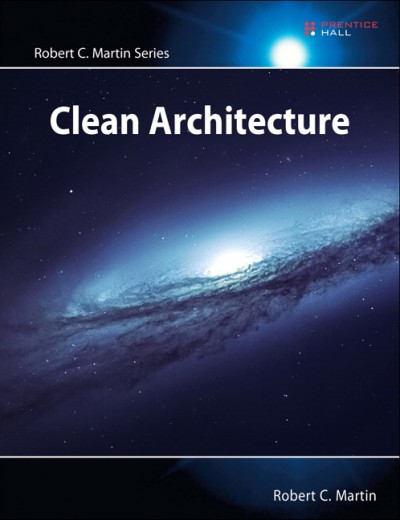
Robert C. Martin
Clean Architecture
A Craftsman's Guide to Software Structure and Design
1. Auflage
Erscheinungsjahr: 2017
Print-ISBN: 978-0-13-449416-6
E-ISBN: 978-0-13-449433-3
Sprache: Englisch
Zusammenfassung
Practical Software Architecture Solutions from the Legendary Robert C. Martin (“Uncle Bob”). By applying universal rules of software architecture, you can dramatically
improve developer productivity throughout the life of any software system. Now, building upon the success of his best-selling books "Clean Code" and "The Clean Coder"
legendary software craftsman Robert C. Martin (“Uncle Bob”) reveals those rules and helps you apply them.
Martin’s "Clean Architecture" doesn’t merely present options. Drawing on over a half-century of experience in software environments of every imaginable type,
Martin tells you what choices to make and why they are critical to your success. As you’ve come to expect from Uncle Bob, this book is packed with direct,
no-nonsense solutions for the real challenges you’ll face–the ones that will make or break your projects.
- Learn what software architects need to achieve–and core disciplines and practices for achieving it.
- Master essential software design principles for addressing function, component separation, and data management
- See how programming paradigms impose discipline by restricting what developers can do
- Understand what’s critically important and what’s merely a “detail”
- Implement optimal, high-level structures for web, database, thick-client, console, and embedded applications
- Define appropriate boundaries and layers, and organize components and services
- See why designs and architectures go wrong, and how to prevent (or fix) these failures
"Clean Architecture" is essential reading for every current or aspiring software architect, systems analyst, system designer, and software manager–and for every programmer who must execute someone else’s designs.
Inhaltsverzeichnis
Über die Autoren
Robert C. Martin
Author