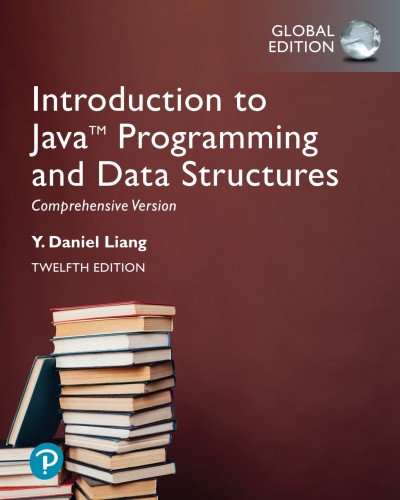
Y. Liang
Introduction to Java Programming and Data Structures, Comprehensive Version
Global Edition
12. Auflage
Erscheinungsjahr: 2021
Print-ISBN: 978-1-292-40207-9
E-ISBN: 978-1-292-40213-0
Seiten: 1240
Sprache: Englisch
Zusammenfassung
This text is intended for a 1-semester CS1 course sequence. The Brief Version contains the first 18 chapters of the Comprehensive Version. The first 13 chapters are appropriate for preparing the AP Computer Science exam. For courses in Java Programming. A fundamentals-first introduction to basic programming concepts and techniques. Designed to support an introductory programming course, Introduction to Java Programming and Data Structuresteaches concepts of problem-solving and object-orientated programming using a fundamentals-first approach. Beginner programmers learn critical problem-solving techniques then move on to grasp the key concepts of object-oriented, GUI programming, data structures, and Web programming. This course approaches Java GUI programming using JavaFX, which has replaced Swing as the new GUI tool for developing cross-platform-rich Internet applications and is simpler to learn and use. The 11th edition has been completely revised to enhance clarity and presentation, and includes new and expanded content, examples, and exercises. Also available with MyLab Programming.MyLab Programming is an online learning system designed to engage students and improve results. MyLab Programming consists of programming exercises correlated to the concepts and objectives in this book. Through practice exercises and immediate, personalized feedback, MyLab Programming improves the programming competence of beginning students who often struggle with the basic concepts of programming languages.
Inhaltsverzeichnis
Über die Autoren
Y. Liang
Author